NFT Gallery is going from strength to strength. One issue I have with it though is the user having to paste their Ethereum address into the wallet page. As anyone can paste any public wallet address, you can have anyones collection on your phone. So I have been thinking how to integrate Web3 tech into the app.
Web3 means cryptocurrencies, blockchain technology and the one problem is that it mostly lives in web browsers. Most people who use Ethereum access it via the Metamask browser plug in. In the mobile world you can’t really have plug ins and so Metamask exists as its own app, Metamask Mobile (or MMM for short). This lets you manage your accounts and interact with Dapps via the built in browser.
So how do you get two apps to talk to each other? The answer is deeplinks. MMM has a couple of deeplinks that allow you to trigger things in the app. One is to open a Dapp (or a website). The trick is to open a webpage in MMM, get the users Eth address and then trigger a deeplink back into your app. Luckily I know a smattering of Javascript and had played with deeplinks on a previous project.
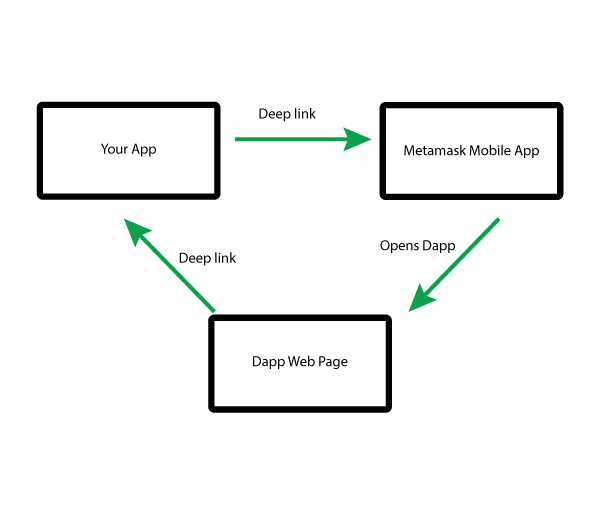
First of all you create a web page on an https web server. Mine is here. The gist of it is that Metamask injects a javascript object called “ethereum” into the web page and the code interacts with that. Debugging it on the desktop was quite easy but in the app, it worked slightly differently. To debug you need to build your own debug version of MMM and remotely connect from the desktop to view your javascript. I got it working though.
To call MMM and get it to run your code you do something like this in c#.
await Browser.OpenAsync($"https://metamask.app.link/dapp/www.redfivesoftware.com/Web3Metamask.htm?return=http://" + AppName);
This opens a url on the device, which opens the deeplink into the app, which in turn opens your web page in the browser tab. In this case Web3Metamask.htm. Currently I pass one parameter in which is the start of the url that calls back to my app.
Then it is simply a case of overriding the OnAppLinkRequestReceived in your App class and processing the URI that was passed back into you.
protected override void OnAppLinkRequestReceived(Uri uri)
{
Logger.Info(uri.AbsolutePath);
string url = uri.AbsolutePath;
var bits= url.Split("/",StringSplitOptions.RemoveEmptyEntries);
switch (bits[0])
{
//{nftgallery:///address/0xce41b49d28800de3ba9925f68d82129dfc4b870b}
case "address":
string address = bits[1];
App.SettingsViewModel.UserAddress = address;
break;
default:
break;
}
base.OnAppLinkRequestReceived(uri);
}
And now you have your eth address. In the next post I will show how to get details of NFTs from OpenSea using their API.